Developing applications for Windows Phone 7.5, codenamed Mango, is very easy. However, it’s good to know a few areas that can confuse a novice developer. The article focuses mainly on background tasks that have their own specifics and uploading applications to the Marketplace. Next, we'll show you what the local Code-First Entity Framework looks like.
Windows Phone layer on top of Windows CE with IE9 and CoreCLR. And because CoreCLR also uses Silverlight, development for WPF, Silverlight or Windows Phone is basically the same. However, what differs significantly is the architecture of the application, which is greatly influenced by the technical specifics of Windows Phone. These are mainly due to the fact that the device is powered by an electric battery.
User interface
In WPF, binding works with all the trimmings and MVVM should be a matter of course. WPF is designed for it. However, Windows Phone has the following limitations:
- In the application menu (
ApplicationBar
), bindig cannot be used. - There is no support for
DependencyPropertyDescriptor
. Command
is missing completely.- The DataContextChanged event is not implemented.
- You can only dream of Style Trigger.
The Dispatcher has moved to Deployment.Current.Dispatcher
. It is important to keep in mind that when a control has defined removing its events in XAML, the name of the handler method must be unique in the sense that there must not be a method that has the same name but has different parameters. More specifically, if a handler is registered in XAML, it must not be overloaded.
Because MVVM can only be used hybridly, not completely, you can't avoid the old approach of enabling or disabling controls. The easiest way is to create a method that sets the individual controls and call it whenever an action has been performed that affects them.
Marketplace
If you have an ISIC, you can sign up for the App Hub for free if you link your ISIC and Windows Live ID to DreamSpark. Before uploading your application to the Marketplace, it is a good idea to prevent the most common errors by running tests in the Tesk Kit Marketplace.
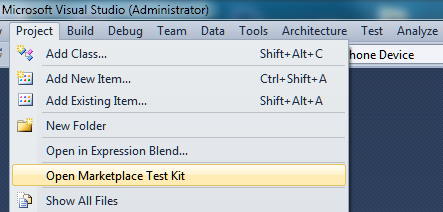
When you upload a private beta test, the app will go through within a few hours, but it takes about a day to promote it on the Czech Marketplace, from where it can only be installed. It is also good to know that the beta version cannot be overwritten by a newer version. It is therefore good to distinguish it in the name of the application, because the name must be unique.
Entity Framework
The on-premises Entity Framework is so similar (Code-First) to Entity Framework 4.3 that I hope Entity Framework 5 will be for both large .NET and CoreCLR. There is a class that represents the database and its properties represent the tables. The classes that represent the tables implement the INotifyPropertyChanged and INotifyPropertyChanging interfaces (to make this easier for EF for performance). The index is defined by an attribute that decorates the class representing the table. Migration is handled by schema versioning.
Scheduled tasks
There are two types of scheduled tasks – Periodic and Resource-Intensive. The first one starts often, but it is very limited in time and memory, the second one only starts when the phone is charging and is within Wi-Fi range. It is necessary to keep this in mind when designing the application and try to do only the essentials in background tasks. Leave the rest of the operations that can wait for the application to start. Registration of scheduled tasks is tricky in that after re-registration, data such as LastSheduledTime
and LastExitReason
disappear.
The threads work normally and are registered via ThreadPool.QueueUserWorkItem
. However, it is not a good idea to register more than one thread at the same time, because it can be noticeable in the response of the user interface. To synchronize threads easily, use AutoResetEvent.
When you execute code in other threads, you need to return to STAThread and make changes to the user interface. When you inherit a ViewModel from this class, you'll already have cross-threading implemented:
public class DispatcherControl : INotifyPropertyChanged {
protected void ExecInForeground(Action lambda) {
if (Thread.CurrentThread.IsBackground) {
Deployment.Current.Dispatcher.BeginInvoke(lambda);
} else {
lambda();
}
}
protected void ExecInBackground<T>(Func<T> lambda, Action<T> finish) {
if (!Thread.CurrentThread.IsBackground) {
ThreadPool.QueueUserWorkItem(e => {
Deployment.Current.Dispatcher.BeginInvoke(finish, lambda());
});
} else {
Deployment.Current.Dispatcher.BeginInvoke(finish, lambda());
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected void NotifyPropertyChanged(String propertyName) {
PropertyChangedEventHandler handler = PropertyChanged;
if (null != handler) {
ExecInForeground(() => handler(this, new PropertyChangedEventArgs(propertyName)));
}
}
}
You do the work in the background and set the individual properties of the ViewModel via STAThred
. This is necessary because setting properties raises the PropertyChanged
event, which is already removed by individual UI controls.
Bookcases
If you don't find enough functionality in the . NET for Windows Phone, you can extend it with libraries from Silverlight, which can be found in C:\Program Files (x86)\Microsoft SDKs\Silverlight\v4.0\Libraries\Client\
. Keep in mind, however, that the main reason these libraries aren't included in your Windows Phone is because they're memory-intensive. They are not usable in the Resource-Intensive task either. Other useful open-source libraries can be found in the App Hub.