Metro style application must contain a massive local cache in order to achieve fast and fluid user experience. Since data could be refreshed only by background task, every data model must be stored in the local application data storage. While I have been writing a code to transfer application data between model classes and local storage I realized I can write a tiny layer doing this job for me automatically. This article describes how to use it.
The architecture is quite simple and familiar for those who develop Windows Phone applications. Data are stored in the database or local files. Database approach derives benefit from Entity Framework. It is simple, effective and robust enough. Alternative approach derives benefit from WinRT object oriented storage. It allows store recurrent structures as well as key and value pairs. But this is not enough. This storage misses the mechanism for storing custom class which contains a collection of another custom class which contains two collections of yet another custom class. I had to code this mechanism during developing one of the first apps for Windows 8.
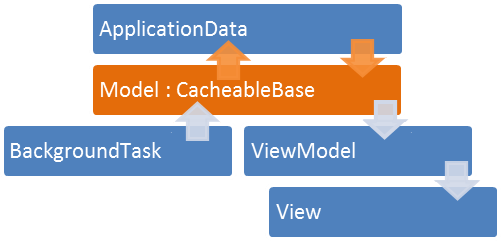
In order to sync model’s data with local application data storage it must derive from the ModelStorageBase
class. This is similar to Entity Framework where the core class is DbContext
. Next, every property must be coded like this:
public class Model : ModelStorageBase {
public Model() : base("Model") { }
public string ScalarProperty {
get { return GetValueProperty<string>(); }
set { SetValueProperty(value); }
}
public ModelStorageReferenceCollection<Item> CollectionProperty {
get { return GetReferenceCollection<Item>(); }
}
}
public class Item : ModelStorageBase {
public Item() : base() { }
public string ScalarProperty {
get { return GetValueProperty<string>(); }
set { SetValueProperty(value); }
}
public ModelStorageValueCollection<int> CollectionProperty {
get { return GetValueCollection<int>(); }
}
}
Constructor defines a key used to store class data. ScalarProperty
uses derived method to save or load its value. *ModelStorageBase
implements INotifyPropertyChanged
so don’t need to care about multicasting property’s change notification event. The CollectionProperty
is a collection that contains items which type is derived from the CacheableBase
. The collection is initialized automatically, so it does not need a setter. The ModelStorageReferenceCollection<T>
type is derived from ObservableCollection<T>
which means you don’t need to worry about change notifications. This type also contains an alternative to Add
method. The AddOrUpdate
method is very useful when you want to update an existing items already contained in collection. This is typically used during background task which updating the data. The CollectionProperty
returns the ModelStorageValueCollection<T>
type which is used when T
is a primitive type.
All classes of this tiny framework are available in my ModelStorage Nuget package. Source code is available on Codeplex. Hope you will find it useful.